Kivyにはスイッチやボタンがいくつかあるのでまとめてみました。
個人的にKivyは使用方法が分かりにくくて、躓くことが多いです。そんな時に他の方も躓くことがないようにまとめています。
ここでは、「Button」「Switch」「Togglebutton」「checkbox」の使用例を紹介しています。
この記事の対象
Button
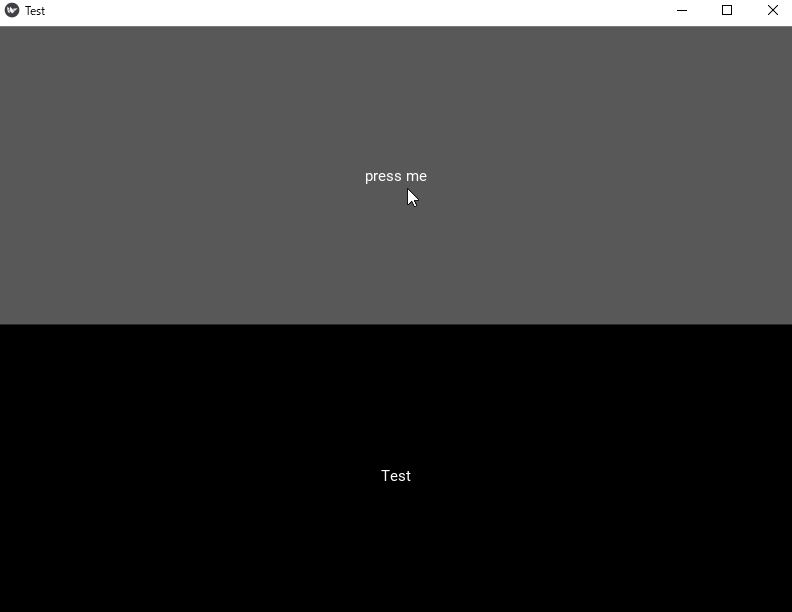
通常のボタン操作です。初期値から押している間の値になって離した時の値に変わる操作が上記になります。Pythonファイルは以下になります。
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.widget import Widget
from kivy.lang import Builder
from kivy.uix.switch import Switch
from kivy.uix.button import Button
import time
class MainScreen(Widget):
def __init__(self, **kwargs):
super().__init__(**kwargs)
def button_clicked(self):
self.ids.lbchange.text = 'Change'
def button_release(self):
self.ids.lbchange.text = 'Release'
class TestApp(App):
def __init__(self, **kwargs):
super(TestApp, self).__init__(**kwargs)
self.title = 'Test'
if __name__ == '__main__':
TestApp().run()
Kvファイルは以下になります。Buttonに「on_press」「on_release」時の関数を指定しています。
<MainScreen>:
BoxLayout:
orientation: 'vertical'
size: root.size
Button:
text: 'press me'
on_press: root.button_clicked()
on_release: root.button_release()
Label:
text: 'Test'
id: lbchange
MainScreen:
Buttonに画像を挿入
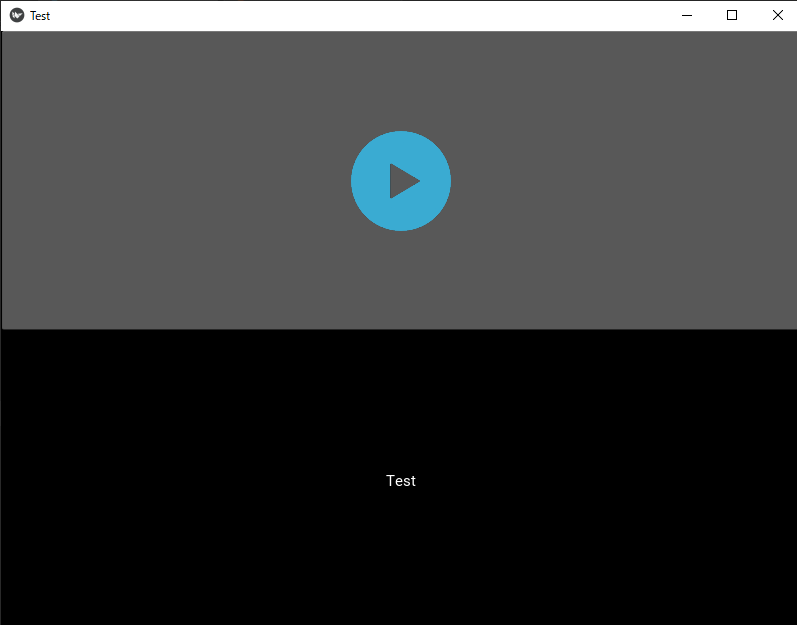
画像を挿入する場合はKVファイルで「Button」の中に配置するだけです。引数としては「source」が必要であり、Pathを指定して下さい。
<MainScreen>:
BoxLayout:
orientation: 'vertical'
size: root.size
Button:
on_press: root.button_clicked()
on_release: root.button_release()
Image:
id: btn_image
source: "button.png"
center_x: self.parent.center_x
center_y: self.parent.center_y
Label:
text: 'Test'
id: lbchange
MainScreen:
Switch
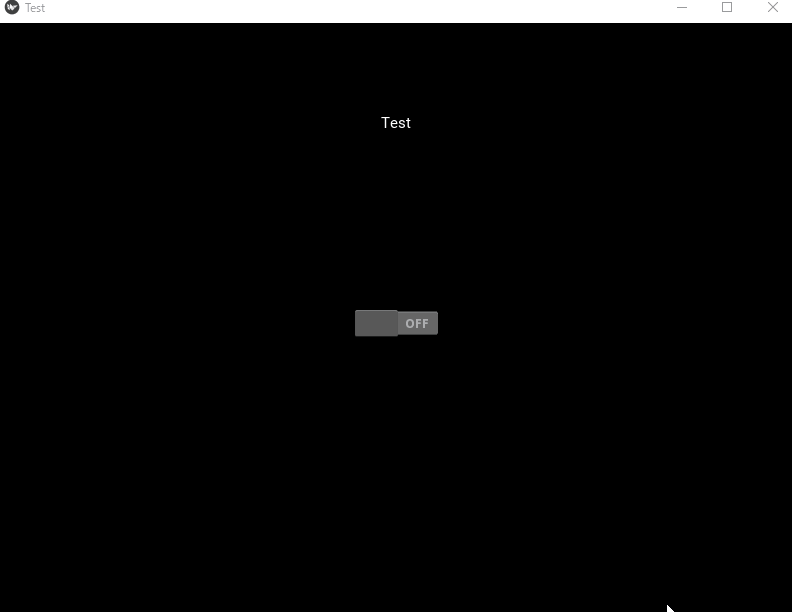
kivyのSwitchはボタンをクリックしてOn/Offできるのとは別にボタンを押しながらスライドもできます。用いたPythonファイルは以下になります。
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.widget import Widget
from kivy.lang import Builder
from kivy.uix.switch import Switch
class MainScreen(Widget):
def __init__(self, **kwargs):
super().__init__(**kwargs)
class TestApp(App):
def __init__(self, **kwargs):
super(TestApp, self).__init__(**kwargs)
self.title = 'Test'
if __name__ == '__main__':
TestApp().run()
Kvファイルは以下になります。
<MainScreen>:
BoxLayout:
orientation: 'vertical'
size: root.size
Label:
text: 'Test'
Switch:
id: switch
Label:
text: 'Succsess!!!!!!!!' if switch.active else ''
MainScreen:
Toggle button
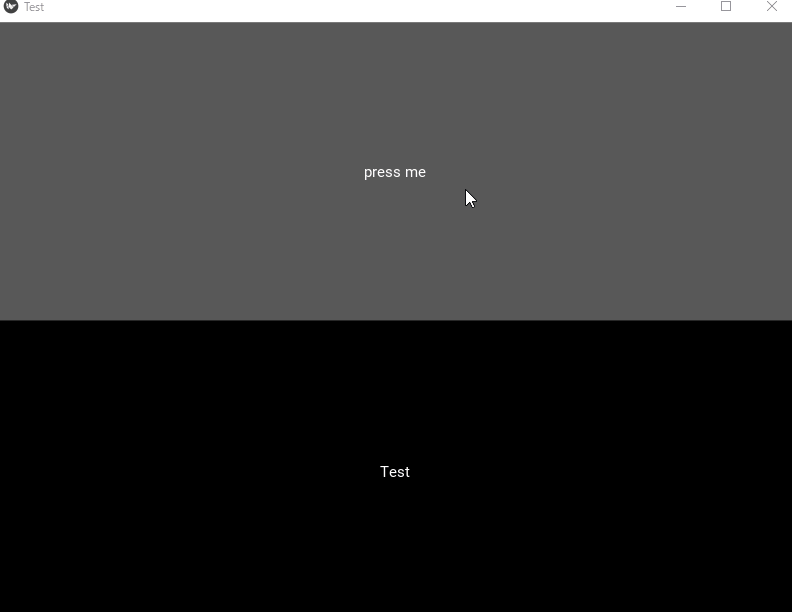
「Toggule Button」は押したらOn/Offの状態が分かるボタンです。「state」変数で押されているか分かります。Pythonファイルは以下になります。
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.widget import Widget
from kivy.lang import Builder
from kivy.uix.switch import Switch
from kivy.uix.button import Button
import time
class MainScreen(Widget):
def __init__(self, **kwargs):
super().__init__(**kwargs)
def button_clicked(self):
self.ids.lbchange.text = 'Change'
def button_release(self):
self.ids.lbchange.text = 'Release'
class TestApp(App):
def __init__(self, **kwargs):
super(TestApp, self).__init__(**kwargs)
self.title = 'Test'
if __name__ == '__main__':
TestApp().run()
Kvファイルは以下になります。
<MainScreen>:
BoxLayout:
orientation: 'vertical'
size: root.size
ToggleButton:
text: 'press me'
on_press: root.button_clicked()
on_release: root.button_release()
Label:
text: 'Test'
id: lbchange
MainScreen:
Checkbox
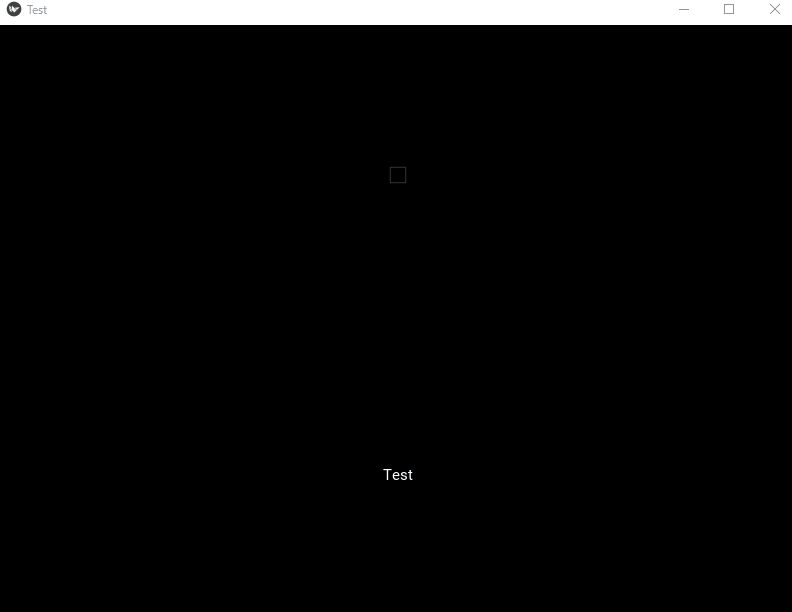
「Checkbox」はToggule Buttonと同じで状態が分かるボタンになります。Pythonファイルは以下になります。
from kivy.app import App
from kivy.uix.label import Label
from kivy.uix.widget import Widget
from kivy.lang import Builder
from kivy.uix.switch import Switch
from kivy.uix.button import Button
class MainScreen(Widget):
def __init__(self, **kwargs):
super().__init__(**kwargs)
def button_clicked(self):
self.ids.lbchange.text = 'Change'
def button_release(self):
self.ids.lbchange.text = 'Release'
class TestApp(App):
def __init__(self, **kwargs):
super(TestApp, self).__init__(**kwargs)
self.title = 'Test'
if __name__ == '__main__':
TestApp().run()
Kvファイルは以下になります。
<MainScreen>:
BoxLayout:
orientation: 'vertical'
size: root.size
CheckBox:
on_press: root.button_clicked()
on_release: root.button_release()
Label:
text: 'Test'
id: lbchange
MainScreen: